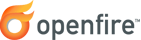
Custom Authentication Provider Guide
Introduction
This document provides instructions on how to implement an integration between Openfire and an external system that provides authentication functionality.
This integration requires some Java knowledge in order to implement a custom authentication provider for Openfire. The skill needed will vary depending on what you are trying to achieve.
Topics that are covered in this document:
Background
Under standard configuration, Openfire maintains authentication data in its own database tables. Various alternatives to this are offered that allow you to use Active Directory or LDAP for authentication or integrating authentication with your custom database tables.
If you're interested in integrating with a system that is not compatible with the standard integration options that are provided by Openfire, then you can implement a custom integration. This guide will help you get started!
It is good to realize that the provider architecture that is used in this guide is never used by end-user clients directly. Instead, the implementation that you will create based on this guide is used by the Openfire service itself. This service will use it to process client authentication requests (which typically use a SASL mechanism). The implementation of custom SASL mechanisms is out of scope of for this guide).
The AuthProvider extension point
Openfire's API defines the AuthProvider interface, which is the extension point to use when implementing custom authentication functionality.
The default implementation of this provider is the DefaultAuthProvider
, which as the name
suggests is the version of this provider Openfire will use if not overridden. It authenticates against the
ofUser
database table and supports plain text and digest authentication.
The steps to get Openfire using a custom AuthProvider
are described below.
-
Write a class that implements
AuthProvider
, providing your own business logic. - Make the class available in a jar and make this available to Openfire by placing it in the lib directory. Why not use an Openfire plugin, you ask? Read the FAQ entry below! There are numerous ways to package a jar with this class inside it, popular build systems such as Gradle and Maven can make your life easier.
-
Set the property
provider.auth.className
to be the full name of your class, e.g.org.example.auth.MyAuthProvider
. You can easily do this by defining such a property in theconf/openfire.xml
configuration file, as shown below. - Restart Openfire. Your custom class should now be handling authentication.
It is worth noting that the AuthProvider
interface defines an interface that can be used to
both read data from, but also write data back to the system that is being integrated with. If the system
that you're integrating with can not or must not have its user definitions changed, you can implement your
provider as being 'read-only'.
To mark your provider as being read-only, implement the isReadOnly()
method to return the value
true
. All methods that would cause a change of data won't be invoked by Openfire if you do so.
These methods should receive an implementation that throws java.lang.UnsupportedOperationException
.
A similar approach (throwing java.lang.UnsupportedOperationException
) can be used to prevent
implementing optional functionality, such as the support for hash/digest-based credentials.
Frequently Asked Questions
Do I have to compile my custom class into the Openfire jar?
No, the class only needs to be visible on the Openfire classpath.
How do I ensure my custom class is visible on the Openfire classpath?
Just place your new custom library in the Openfire lib directory, this will ensure it is automatically available at startup.
Should I include all the dependencies that my code needs?
Yes, and no. Your code will run using the same classpath as Openfire. Openfire's dependencies are already on that classpath. If your code uses the same dependencies, you do not need to include them again. You can add other dependencies, either by packaging them in your jar file, or by placing them in the openfire lib folder.
Beware of conflicting dependencies! Sometimes, packaging dependencies in an all-containing jar, and renaming the packages of those dependencies (shade them), can be helpful.
Isn't it easier to deploy my code as an Openfire plugin?
It is not recommended to use a plugin for packaging and deploying your provider. For one, plugins use a dedicated class path. For another, the load order of plugins could lead to hairy scenarios, and finally: plugins can be unloaded at runtime. This all could lead to a running Openfire service for which you cannot guarantee that your provider is loaded at all times. For these reasons, we recommend creating a jar file instead of an Openfire plugin, and placing that jar file in Openfire's lib directory!
Can I see some examples?
Openfire's own authentication mechanism makes use of the AuthProvider
API! If you want to get
some inspiration, you can have a look at the implementations of this interface that are part of Openfire,
such as the ones below.
org.jivesoftware.openfire.auth.DefaultAuthProvider
- used as the default provider.org.jivesoftware.openfire.auth.JDBCAuthProvider
- integrates with a custom database.org.jivesoftware.openfire.ldap.LdapAuthProvider
- used when Openfire is configured to integrate with Active Directory or LDAP.
Note that these providers are but a sample of the available providers. Discover more providers by using your IDE to find implementations of the interface!
Will I have a degradation in performance using a custom AuthProvider?
It completely depends on your implementation. As with any Openfire customisation or plugin, badly written code has the potential to cause Openfire to perform slower. Use performance testing tools such as Tsung to ensure issues haven't been introduced.
How can I have my custom class connect to another DB/Web service/NoSQL store etc?
This is out of the scope of this documentation and is your choice as a developer. If you are looking to externalize properties like connection details, the Openfire properties mechanism and the JiveGlobals class are good places to start investigating.