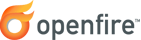
Twisted Words: A Minimal Working Example (in Python)
Introduction
This document provides a minimal working example of a client implementation using the Twisted Words library, making it connect to a running Openfire server.
Topics that are covered in this document:
Background
Twisted is an event-based framework for internet applications, supporting Python 3.6+. It includes modules for many different purposes, including the Words module, that adds support for the XMPP protocol.
This guide describes how to use Twisted Words to connect to Openfire. It provides nothing more than a minimal working example, intended as a stepping stone to for client developers that get started with a new project.
Preparations
In this example, a client connection will be made against a running Openfire server. For ease of configuration, the 'demoboot' setup of Openfire is used.
The 'demoboot' setup of Openfire allows one to start a fresh installation of Openfire into a certain provisioned state, without running any of the setup steps. When running in 'demoboot' mode:
- an administrative account is created using the username 'admin' and password 'admin'
- two users are automatically created: 'jane' and 'john' (both using the value 'secret' as their password)
- the XMPP domain name is configured to be 'example.org' (for ease of use, configure 'example.org' to be an alias of '127.0.0.1' in your hosts file!)
To start Openfire in 'demoboot' mode, you can invoke the Openfire executable using the -demoboot
argument, as shown below.
Code
First, create the Twisted module for Python, as shown below.
Create a file named xmpp_client.py
and copy the code shown below.
Execute the example client, and provide the JID of 'john' as well as it's password, as shown below:
If all goes well, this will print a short exchange of XMPP data.
Note that this example is based on Twisted's example code, but modified to disable important security features. You should not use this for anything important!
Further Reading
Please use the links below to find more information.