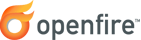
Strophe.js: A Minimal Working Example (in Javascript)
Introduction
This document provides a minimal working example of a client implementation using the Strophe.js library, making it connect to a running Openfire server.
Topics that are covered in this document:
Background
Strophe.js is an XMPP library for JavaScript. Its primary purpose is to enable web-based, real-time XMPP applications that run in any browser.
This guide describes how to use Strophe.js to connect to Openfire. It provides nothing more than a minimal working example, intended as a stepping stone to for client developers that get started with a new project.
Preparations
In this example, a client connection will be made against a running Openfire server. For ease of configuration, the 'demoboot' setup of Openfire is used.
The 'demoboot' setup of Openfire allows one to start a fresh installation of Openfire into a certain provisioned state, without running any of the setup steps. When running in 'demoboot' mode:
- an administrative account is created using the username 'admin' and password 'admin'
- two users are automatically created: 'jane' and 'john' (both using the value 'secret' as their password)
- the XMPP domain name is configured to be 'example.org' (for ease of use, configure 'example.org' to be an alias of '127.0.0.1' in your hosts file!)
To start Openfire in 'demoboot' mode, you can invoke the Openfire executable using the -demoboot
argument, as shown below.
Code
Download and build the Strophe.js distribution. Assuming that you have a compatible version of Nodejs installed, this can be as simple as executing the 'dist' make target, as shown below, in the directory in which the Strophe.js release archive was extracted.
If the build is successful, several files will have been created in a directory called dist
. In
this example, the file dist/strophe.umd.js
will be used.
Find (or create) the directory examples
in the directory in which the Strophe.js release
archive was extracted (it should be a sibling to the dist
directory that was created). In the
exmples
directory, create a file called openfire.html
and copy the code shown
below.
Save the file, and open it in a browser. You should be presented with two input fields. Fill out the
password for the username john
(it's: secret
) and press the 'connect' button. You
will immediately see all raw XMPP that is being exchanged with the server!
Note that this example disables important security features. You should not use this for anything important!
Further Reading
Please use the links below to find more information.