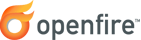
StanzaJS: A Minimal Working Example (in Javascript/Typescript)
Introduction
This document provides a minimal working example of a client implementation using the StanzaJS library, making it connect to a running Openfire server.
Topics that are covered in this document:
Background
StanzaJS is a JavaScript/TypeScript library for using modern XMPP, and it does that by exposing everything as JSON. Unless you insist, you have no need to ever see or touch any XML when using StanzaJS.
This guide describes how to use StanzaJS to connect to Openfire. It provides nothing more than a minimal working example, intended as a stepping stone to for client developers that get started with a new project.
Preparations
In this example, a client connection will be made against a running Openfire server. For ease of configuration, the 'demoboot' setup of Openfire is used.
The 'demoboot' setup of Openfire allows one to start a fresh installation of Openfire into a certain provisioned state, without running any of the setup steps. When running in 'demoboot' mode:
- an administrative account is created using the username 'admin' and password 'admin'
- two users are automatically created: 'jane' and 'john' (both using the value 'secret' as their password)
- the XMPP domain name is configured to be 'example.org' (for ease of use, configure 'example.org' to be an alias of '127.0.0.1' in your hosts file!)
To start Openfire in 'demoboot' mode, you can invoke the Openfire executable using the -demoboot
argument, as shown below.
Code
Copy the following code in a fresh project.
When this project is running, have a look at the Openfire Administrative Console: you should notice that the user named 'john' is online! Use any other XMPP client to log in with the user 'jane', and send a message to John. The code above will cause John to echo back the message that you have sent.
Note that this example disables important security features. You should not use this for anything important!
Further Reading
Please use the links below to find more information.