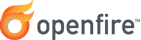
Mellium: A Minimal Working Example (in Go)
Introduction
This document provides a minimal working example of a client implementation using the Mellium library, making it connect to a running Openfire server.
Topics that are covered in this document:
Background
Mellium provides a collection of Go libraries, tools, and applications related to XMPP and the Jabber network.
This guide describes how to use Mellium to connect to Openfire. It provides nothing more than a minimal working example, intended as a stepping stone to for client developers that get started with a new project.
Preparations
In this example, a client connection will be made against a running Openfire server. For ease of configuration, the 'demoboot' setup of Openfire is used.
The 'demoboot' setup of Openfire allows one to start a fresh installation of Openfire into a certain provisioned state, without running any of the setup steps. When running in 'demoboot' mode:
- an administrative account is created using the username 'admin' and password 'admin'
- two users are automatically created: 'jane' and 'john' (both using the value 'secret' as their password)
- the XMPP domain name is configured to be 'example.org' (for ease of use, configure 'example.org' to be an alias of '127.0.0.1' in your hosts file!)
To start Openfire in 'demoboot' mode, you can invoke the Openfire executable using the -demoboot
argument, as shown below.
That should be everything that you need to get Openfire running. Background information on the 'demoboot' mode can be found in Openfire's Demoboot Guide.
Code
To start the project, create a file named test.go
in an empty directory, and copy in the code
below.
Finally, build and run the test client, using the instructions below.
You should see the raw XMPP exchange with the server, ending with a closing stream element.
Note that this example disables important security features. You should not use this for anything important!
Further Reading
Please use the links below to find more information.